Assignment 6B: Maze Game – Version 2! In the "real world", you rarely write a piece of software once and then leave it. Instead, you make updates and fixes as you improve as a programmer. In this assignment, we are going to take our code from Assignment 5B and make the following code improvements with methods. Note for C++ Students: To use a 2D array as a parameter, you need to include the numbers of columns in the method header. For example, “void setArray (int arr[][5])" would accept a 5x5 2D array. - Create a method called checkValidPosition. It should take in as parameters the current 2D array and two ints – one that represents the new X position and one that represents the new Y position of the Player. It should determine if the new position is valid (within the bounds of the array), and return a boolean TRUE or FALSE to the Main method. This function should be used to replace the code that was previously used to do this work in your code from Assignment 5B. Create a method called checkGameStatus. It should take in as parameters the current 2D array and two ints that represent the X/Y position of the player. It should determine if the player is on the path or has won or lost the game. It should return an integer to the Main method that represents each state: o Returning 0 means the player has lost o Returning 1 means the player is still playing the game o Returning 2 means the player has won the game. This function should be used to replace the code that was previously used to do this work in your code from Assignment 5B. Create a method called printMaze. It should take in as parameters the current 2D array. It should print the maze to the screen; it should not return any value to the Main method. This function should be used to replace the code that was previously used to do this work in your code from Assignment 5B.
Assignment 6B: Maze Game – Version 2! In the "real world", you rarely write a piece of software once and then leave it. Instead, you make updates and fixes as you improve as a programmer. In this assignment, we are going to take our code from Assignment 5B and make the following code improvements with methods. Note for C++ Students: To use a 2D array as a parameter, you need to include the numbers of columns in the method header. For example, “void setArray (int arr[][5])" would accept a 5x5 2D array. - Create a method called checkValidPosition. It should take in as parameters the current 2D array and two ints – one that represents the new X position and one that represents the new Y position of the Player. It should determine if the new position is valid (within the bounds of the array), and return a boolean TRUE or FALSE to the Main method. This function should be used to replace the code that was previously used to do this work in your code from Assignment 5B. Create a method called checkGameStatus. It should take in as parameters the current 2D array and two ints that represent the X/Y position of the player. It should determine if the player is on the path or has won or lost the game. It should return an integer to the Main method that represents each state: o Returning 0 means the player has lost o Returning 1 means the player is still playing the game o Returning 2 means the player has won the game. This function should be used to replace the code that was previously used to do this work in your code from Assignment 5B. Create a method called printMaze. It should take in as parameters the current 2D array. It should print the maze to the screen; it should not return any value to the Main method. This function should be used to replace the code that was previously used to do this work in your code from Assignment 5B.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Using Assignment 5B and turning it into assignment 6B using c++ language
![Assignment 5B: Maze Game! 2D Arrays can be used to store and represent information
about video game levels or boards. In this exercise, you will use this knowledge to
create an interactive game where players attempt to move through a maze. You will
start by creating a pre-defined 2D array with the following values:
"X","X"}
X","W"}
You will then set the player (represented by "O") at index 0, O of the array, the top-left
corner of the maze. You will use a loop to repeatedly prompt the user to enter a
direction ("Left", "Right", "Up", or "Down"). Based on these directions, you will try to
move the player.
If the location is valid (represented by "_"), you will move the player there
If the location is out of bounds (e.g. index 0, -1) or the command is invalid, you
will inform the player and prompt them to enter another direction
• f the location is a wall (represented by "X"), you will tell the user they hit a wall
and the game is over.
If the player did not lose, you will print out the current state of the map and ask them for
a new direction. You will keep doing this until they lose or they reach the end
(represented by the "W").
Sample Output #1:
[Maze Game]
0.Х._.х.х.
-X._.X.W.
---X._.
X.X.
---X.X.
Which direction do you want to move? Up
You can't move there - it's out of bounds!
Which direction do you want to move? Down
--Х. .х.х.
0.Х._.Х.W.
----X._.
X.X.--
.X.X.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe799f64b-1f0b-4ff1-9ee3-5090ddf07729%2Fa4501d6c-9251-4a53-97fd-9136046ab7e0%2Fze2kjy_processed.png&w=3840&q=75)
Transcribed Image Text:Assignment 5B: Maze Game! 2D Arrays can be used to store and represent information
about video game levels or boards. In this exercise, you will use this knowledge to
create an interactive game where players attempt to move through a maze. You will
start by creating a pre-defined 2D array with the following values:
"X","X"}
X","W"}
You will then set the player (represented by "O") at index 0, O of the array, the top-left
corner of the maze. You will use a loop to repeatedly prompt the user to enter a
direction ("Left", "Right", "Up", or "Down"). Based on these directions, you will try to
move the player.
If the location is valid (represented by "_"), you will move the player there
If the location is out of bounds (e.g. index 0, -1) or the command is invalid, you
will inform the player and prompt them to enter another direction
• f the location is a wall (represented by "X"), you will tell the user they hit a wall
and the game is over.
If the player did not lose, you will print out the current state of the map and ask them for
a new direction. You will keep doing this until they lose or they reach the end
(represented by the "W").
Sample Output #1:
[Maze Game]
0.Х._.х.х.
-X._.X.W.
---X._.
X.X.
---X.X.
Which direction do you want to move? Up
You can't move there - it's out of bounds!
Which direction do you want to move? Down
--Х. .х.х.
0.Х._.Х.W.
----X._.
X.X.--
.X.X.
![Assignment 6B: Maze Game – Version 2! In the "real world", you rarely write a piece
of software once and then leave it. Instead, you make updates and fixes as you improve
as a programmer. In this assignment, we are going to take our code from Assignment
5B and make the following code improvements with methods.
Note for C++ Students: To use a 2D array as a parameter, you need to include the
numbers of columns in the method header. For example, "void setArray (int arr[][5])"
would accept a 5x5 2D array.
Create a method called checkValidPosition. It should take in as parameters the
current 2D array and two ints – one that represents the new X position and one
that represents the new Y position of the Player. It should determine if the new
position is valid (within the bounds of the array), and return a boolean TRUE or
FALSE to the Main method.
This function should be used to replace the code that was previously used to do
this work in your code from Assignment 5B.
Create a method called checkGameStatus. It should take in as parameters the
current 2D array and two ints that represent the X/Y position of the player. It
should determine if the player is on the path or has won or lost the game. It
should return an integer to the Main method that represents each state:
o Returning 0 means the player has lost
o Returning 1 means the player is still playing the game
o Returning 2 means the player has won the game.
This function should be used to replace the code that was previously used to do
this work in your code from Assignment 5B.
Create a method called printMaze. It should take in as parameters the current
2D array. It should print the maze to the screen; it should not return any value to
the Main method.
This function should be used to replace the code that was previously used to do
this work in your code from Assignment 5B.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe799f64b-1f0b-4ff1-9ee3-5090ddf07729%2Fa4501d6c-9251-4a53-97fd-9136046ab7e0%2Frv6hmeh_processed.png&w=3840&q=75)
Transcribed Image Text:Assignment 6B: Maze Game – Version 2! In the "real world", you rarely write a piece
of software once and then leave it. Instead, you make updates and fixes as you improve
as a programmer. In this assignment, we are going to take our code from Assignment
5B and make the following code improvements with methods.
Note for C++ Students: To use a 2D array as a parameter, you need to include the
numbers of columns in the method header. For example, "void setArray (int arr[][5])"
would accept a 5x5 2D array.
Create a method called checkValidPosition. It should take in as parameters the
current 2D array and two ints – one that represents the new X position and one
that represents the new Y position of the Player. It should determine if the new
position is valid (within the bounds of the array), and return a boolean TRUE or
FALSE to the Main method.
This function should be used to replace the code that was previously used to do
this work in your code from Assignment 5B.
Create a method called checkGameStatus. It should take in as parameters the
current 2D array and two ints that represent the X/Y position of the player. It
should determine if the player is on the path or has won or lost the game. It
should return an integer to the Main method that represents each state:
o Returning 0 means the player has lost
o Returning 1 means the player is still playing the game
o Returning 2 means the player has won the game.
This function should be used to replace the code that was previously used to do
this work in your code from Assignment 5B.
Create a method called printMaze. It should take in as parameters the current
2D array. It should print the maze to the screen; it should not return any value to
the Main method.
This function should be used to replace the code that was previously used to do
this work in your code from Assignment 5B.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 9 images

Recommended textbooks for you
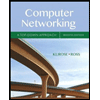
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
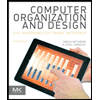
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
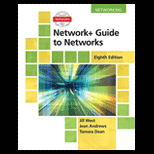
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
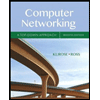
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
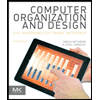
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
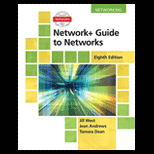
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
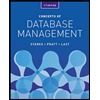
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
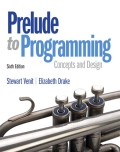
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
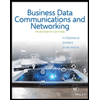
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY