Description In this series of exercises, you will create functions to create, modify and examine dictionaries that represent characters in an animated film, and the cast members who voice the characters. The keys of the dictionary will be character names. The values in the dictionary will be voice actor names. For this exercise, you will create a function that checks if a character is already in the dictionary. Files • monsterfunctions.py : set of functions to work with monster cast dictionaries. Function Name has_character Parameters • monsters: a dictionary • character: a string, the name of a character Action Checks if character is a key in monsters. Return Value True if character is a key in monsters, otherwise False. Examples monsters = create_monster_cast() monsters = add_cast_member(monsters, "Mike", "William Crystal") has_character(monsters, "Mike") -> True has_character(monsters, "Sully") -> False
Exercise: Check Monster Character Exists
Description
In this series of exercises, you will create functions
to create, modify and examine dictionaries that
represent characters in an animated film, and the
cast members who voice the characters. The
keys of the dictionary will be character names.
The values in the dictionary will be voice actor
names.
For this exercise, you will create a function that
checks if a character is already in the dictionary.
Files
• monsterfunctions.py : set of functions to work with monster cast dictionaries.
Function Name
has_character
Parameters
• monsters: a dictionary
• character: a string, the name of a character
Action
Checks if character is a key in monsters.
Return Value
True if character is a key in monsters, otherwise False.
Examples
monsters = create_monster_cast()
monsters = add_cast_member(monsters, "Mike", "William Crystal")
has_character(monsters, "Mike") -> True
has_character(monsters, "Sully") -> False
![# This function creates a dictionary
2 def create_monster_cast():
d1 = {}
1
3
4
return d1
5
6 # This function adds the monsters to the dictionary
7 def add_cast_member (monsters, character, cast_member):
dictionary = monsters
dictionary [character] = cast_member
return dictionary
9
10
11
12 # This function returns the name of cast member associated with the character
13 def get_cast_member(monsters, character):
14
if character not in monsters:
15
return
16
return monsters [character]
17
# This function returns the size of the dictionary
19 # i.e. number of character present in the dictionary
def get_cast_size(monsters):
return len(monsters)
18
20
21
22
23 # Definition of the function to change the cast_member of a
24 def change_cast_member (monsters, character, cast_member):
cter
25
# This statement changes the cast_member
# If the character is not available in the dictionary, then it creates it
monsters[character] = cast_member
26
27
28
29
30
# return the monsters dictionary
31
return monsters
32
33 monsters = create_monster_cast()
34
35 monsters = add_cast_member(monsters, 'James P. "Sulley" Sullivan', "John Goodman")
36 monsters = add_cast_member (monsters, 'Michael "Mike" Wazowski', 'Bill Crystal')
37 monsters = add_cast_member(monsters, 'Boo', 'Mary Gibbs')
38 monsters = add_cast_member(monsters, 'Randall Boggs', 'Steve Buscemi')
39 monsters = add_cast_member(monsters, 'Randall Boggs', 'Steve Buscemi')
40 monsters = add_cast_member(monsters, "Henry J. Waternoose III", 'James Coburn')
monsters = add_cast_member(monsters, "Mike", "William Crystal")
41
42
43
44 print('Monster Cast : ')
45 for key in monsters.keys():
46
print (format(key,"<30"),': ',end='')
print(get_cast_member(monsters, key))
47
48
49
50 # Calling the change_cast_member function with new cast_member value
51 monsters = change_cast_member(monsters, "Mike", "Billy Crystal")
52 print('\nAfter changing the value\n\nMonster Cast : ')
53 for key in monsters.keys():
54
print (format(key,"<30"),': ', end='')
print(get_cast_member(monsters, key))
55
56
57](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe8d8ac33-4e74-4eaf-ac95-0eee83804ad6%2F91f26a46-d86a-4372-b677-7304172f7c43%2Favh1hvd_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

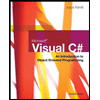
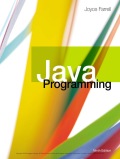
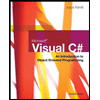
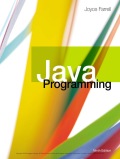