Examples For examples 1 to 4, you must create the data files with a text tool, such as Notepad exampleOne This program reads data from a physical file, create a reference to the file, perform a computation, display the result, close the file package chario; import java.util.Scanner; import java.io.*; // public class Chario { // public static void main(String[] args) throws IOException { File file1 = new File("fileOne.txt"); //Create a reference to the physical file /* Use Notepad to create a text file named fileOne.txt which must be in the same directory that src is in To find src, open your project in NetBeans and hover its name, which is in the top left just below the Projects menu; this will display the path to your project My project path is, yours will be different I:\\Ajava\161\WPPractice\IO\charstream\chario My fileOne.txt is located in chario */ Scanner getit = new Scanner( file1 ); // connect a Scanner to the file int num, square; num = getit.nextInt(); square = num * num ; System.out.println("The square of " + num + " is " + square); getit.close(); //Close the stream } } /* This is not part of your Java code but the file your code will read Your path will be different from mine Create fileOne.txt in I:\\Ajava\161\WPPractice\IO\charstream\chario Type an integer Save fileOne.txt Execute your program from NetBeans */ ExampleTwo This program loops through fileLoop.txt, computation, and display results package charioloop; import java.util.Scanner; import java.io.*; // public class Charioloop { public static void main(String[] args) throws IOException { //Create a reference to the physical file File loopfile = new File("fileLoop.txt"); // connect a Scanner to the file Scanner getAll = new Scanner( loopfile ); int num = 0, square = 0; while(num != -1){ num = getAll.nextInt(); square = num * num ; System.out.println("The square of " + num + " is " + square); } getAll.close(); } } /* Create fileLoop.txt in I:\\Ajava\161\WPPractice\IO\charstream\charioloop My fileLoop.txt looks like this: 2 3 2 10 -1 */ exampleThree In the while loop, this program reads fileLoop.txt until all items are read package hasnext; import java.util.Scanner; import java.io.*; // public class Hasnext { public static void main(String[] args) throws IOException { File loopfile = new File("fileLoop.txt"); Scanner getAll = new Scanner( loopfile ); // connect a Scanner to the file int num = 0, square = 0; while(getAll.hasNextInt()) { num = getAll.nextInt(); square = num * num ; System.out.println("The square of " + num + " is " + square); } getAll.close(); } } exampleFour In the while loop, this program reads fileLoop.txt until all items are read; Each item in the file is stored into an array package storeintoarr; import java.util.Scanner; import java.io.*; public class Storeintoarr { public static void main(String[] args) throws IOException { File loopfile = new File("fileLoop.txt"); Scanner getAll = new Scanner( loopfile ); // connect a Scanner to the file int num = 0, square = 0; int[] items = new int[10]; int i = 0; while(getAll.hasNextInt()){ num = getAll.nextInt(); square = num * num ; //System.out.println("The square of " + num + " is " + square); items[i] = num; System.out.println("items[i] is " + items[i]); i = i + 1; } getAll.close(); } } exampleFive This program creates the file tooutfile.txt in the folder as described in exampleOne Please verify that the file was created package pwriter; import java.util.Scanner; import java.io.*; public class Pwriter { public static void main(String[] args) throws IOException { PrintWriter tofile = new PrintWriter( "tooutfile.txt" ); tofile.println( "1" ); tofile.close(); } } exampleSix This program creates file tooutfile2.txt and stores 10 integers in it Please verify that the file was created along with the content package pwriter2; import java.util.Scanner; import java.io.*; public class Pwriter2 { public static void main(String[] args) throws IOException { PrintWriter tofile2 = new PrintWriter( "tooutfile2.txt" ); int i = 0; while (i < 10){ tofile2.println( i ); i = i + 1; } tofile2.close(); } }
Please help me with example five, thank you!
Examples
For examples 1 to 4, you must create the data files with a text tool, such as Notepad
exampleOne
This
from a physical file,
create a reference to the file,
perform a computation,
display the result,
close the file
package chario;
import java.util.Scanner;
import java.io.*;
//
public class Chario {
//
public static void main(String[] args) throws IOException
{
File file1 = new File("fileOne.txt"); //Create a reference to the physical file
/*
Use Notepad to create a text file named fileOne.txt
which must be in the same directory that src is in
To find src, open your project in NetBeans and hover its name, which is in the top left just below the Projects menu; this will display the path to your project
My project path is, yours will be different
I:\\Ajava\161\WPPractice\IO\charstream\chario
My fileOne.txt is located in chario
*/
Scanner getit = new Scanner( file1 ); // connect a Scanner to the file
int num, square;
num = getit.nextInt();
square = num * num ;
System.out.println("The square of " + num + " is " + square);
getit.close(); //Close the stream
}
}
/*
This is not part of your Java code but the file your code will read
Your path will be different from mine
Create fileOne.txt in
I:\\Ajava\161\WPPractice\IO\charstream\chario
Type an integer
Save fileOne.txt
Execute your program from NetBeans
*/
ExampleTwo
This program loops through fileLoop.txt, computation, and display results
package charioloop;
import java.util.Scanner;
import java.io.*;
//
public class Charioloop {
public static void main(String[] args) throws IOException
{
//Create a reference to the physical file
File loopfile = new File("fileLoop.txt");
// connect a Scanner to the file
Scanner getAll = new Scanner( loopfile );
int num = 0, square = 0;
while(num != -1){
num = getAll.nextInt();
square = num * num ;
System.out.println("The square of " + num + " is " + square);
}
getAll.close();
}
}
/*
Create fileLoop.txt in
I:\\Ajava\161\WPPractice\IO\charstream\charioloop
My fileLoop.txt looks like this:
2
3
2
10
-1
*/
exampleThree
In the while loop, this program reads fileLoop.txt until all items are read
package hasnext;
import java.util.Scanner;
import java.io.*;
//
public class Hasnext {
public static void main(String[] args) throws IOException
{
File loopfile = new File("fileLoop.txt");
Scanner getAll = new Scanner( loopfile );
// connect a Scanner to the file
int num = 0, square = 0;
while(getAll.hasNextInt())
{
num = getAll.nextInt();
square = num * num ;
System.out.println("The square of " + num + " is " + square);
}
getAll.close();
}
}
exampleFour
In the while loop, this program reads fileLoop.txt until all items are read;
Each item in the file is stored into an array
package storeintoarr;
import java.util.Scanner;
import java.io.*;
public class Storeintoarr {
public static void main(String[] args) throws IOException
{
File loopfile = new File("fileLoop.txt");
Scanner getAll = new Scanner( loopfile );
// connect a Scanner to the file
int num = 0, square = 0;
int[] items = new int[10];
int i = 0;
while(getAll.hasNextInt()){
num = getAll.nextInt();
square = num * num ;
//System.out.println("The square of " + num + " is " + square);
items[i] = num;
System.out.println("items[i] is " + items[i]);
i = i + 1;
}
getAll.close();
}
}
exampleFive
This program creates the file tooutfile.txt in the folder as described in exampleOne
Please verify that the file was created
package pwriter;
import java.util.Scanner;
import java.io.*;
public class Pwriter {
public static void main(String[] args) throws IOException
{
PrintWriter tofile = new PrintWriter( "tooutfile.txt" );
tofile.println( "1" );
tofile.close();
}
}
exampleSix
This program creates file tooutfile2.txt and stores 10 integers in it
Please verify that the file was created along with the content
package pwriter2;
import java.util.Scanner;
import java.io.*;
public class Pwriter2 {
public static void main(String[] args) throws IOException
{
PrintWriter tofile2 = new PrintWriter( "tooutfile2.txt" );
int i = 0;
while (i < 10){
tofile2.println( i );
i = i + 1;
}
tofile2.close();
}
}

Step by step
Solved in 2 steps

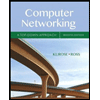
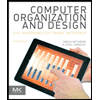
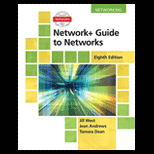
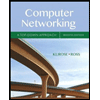
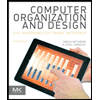
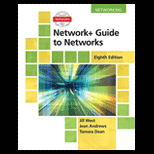
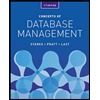
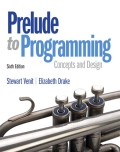
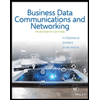