For a lot of the scoring in this game, it is necessary to know the number of times that each number (1 to 6) appears on the dice, and what the maximum number of times is (“3 of a kind", “4 of a kind", etc.). Start with the supplied file Lab11BronzeTemplate.pde and add the following two small functions, in the place indicated. You will also need the two Bronze functions from Lab 10. 1. Write a function int[] freqCount(int[] dice) which will accept an array representing the numbers on the dice (an array of NUM_DICE values, each from 1 to NUM_SIDES). It should create and return an array of NUM_SIDES elements indicating how many times each number from 1 to NUM_SIDES appeared. For example, freqCount(new int[]{1,6,2,1,4}) should return {2,1,0,1,0,1}. Note that since the indices of an array always start at 0, but dice values start at 1, the number of 4's would be at index [3]. 2. Write a function int maxOfAKind(int[] freqs) which accepts any array of integers, and returns the biggest one. In terms of the game, if you give it the frequency count from freqCount, it will tell you how many “of a kind" you have. For example, maxOfAKind(new int[]{0,1,3,0,1,1}) should return 3. The supplied template will allow you to roll 5 dice, and then it will test the above two functions on the 5 dice obtained, printing the results that they give. For example, if you rolled the dice 1-4-3-4-4 the program will print Frequencies of 1..6 are: 1 0 1 3 0 0 (one 1, one 3, three 4's – this is the array freqCount should return) (this is what max0fAKind should give) Largest one is 3 of a kind. You can increase the number of dice (say from 5 to 10) to get a better test.. Your functions should both still work.
Language: Processing
//Constants controlling the game.
//The number of sides should not be changed since the
//graphics only handles from 1-6 dots.
//In this version, the number of dice could be varied.
final int NUM_SIDES=6; //Sides on the dice
final int NUM_DICE=5; //The number of dice used
//-------- Lab 10 Bronze -------------------------------------
//*****INSERT YOUR LAB 10 BRONZE CODE HERE*****
bronze code attached as photo due to word count
//***** ALSO CHANGE mouseClicked() IN THE PLACE INDICATED *****
//***** TO MATCH THE NAME OF YOUR DICE ARRAY VARIABLE *****
//-------- Lab 11 Bronze -------------------------------------
int[] freqCount(int[] roll){
//Count the frequencies of each number (1..NUM_SIDES) in
//the given dice roll, returning the result as a array of
//NUM_SIDES integers. Note that freqs[0..5] are the frequencies
//of 1..6. The dice show 1-6 but subscripts must go 0-5.
}//freqCount
int maxOfAKind(int[] freqs){
//Find and return the largest integer in an array of integers.
}//maxOfAKind
//==================================================================
//
// SUPPLIED CODE - DO NOT EDIT ANYTHING BELOW THIS LINE
void setup(){
size(500,500);
displayMessage("Click to roll");
}//setup
//--------------------------------------------------------------
void draw(){
}//draw
//--------------------------------------------------------------
void mouseClicked(){
background(192);
rollDice();
showDiceRoll();
displayStats(theDice); //*****CHANGE TO THE NAME OF YOUR VARIABLE
}
//--------------------------------------------------------------
void displayMessage(String message){
//Display the given message in the centre of the window.
final int TEXT_SIZE = 20;
textSize(TEXT_SIZE);
fill(0);
//Allow for multi-line messages. Count the \n characters to see.
int numLines = 1; //One by default
for(int i=0; i<message.length(); i++)
if(message.charAt(i) == '\n')
numLines++; //Add one more line for every \n character found
text(message,(width-textWidth(message))/2,
height/2-TEXT_SIZE*numLines/2);
}
//--------------------------------------------------------------
void displayStats(int[] theDice){
//Test the freqCount and maxOfAKind functions by calling them
//and displaying the results as a message.
int[] freqs = freqCount(theDice);
int maxFreq = maxOfAKind(freqs);
String message = "Frequencies of 1.."+NUM_SIDES+" are:\n";
for(int i=0; i<NUM_SIDES; i++)
message += freqs[i]+" ";
message += "\nLargest one is "+maxFreq+" of a kind.\n";
message += "Click to roll again.";
displayMessage(message);
}
//--------------------------------------------------------------
void drawDie(int position, int value){
/* Draw one die in thecanvas.
* **This will only work for dice with up to 6 sides.**
* position - must be 0..NUM_DICE-1, indicating which die is being drawn
* value - must be 1..6, the amount showing on that die
*/
final float X_SPACING = (float)width/NUM_DICE; //X spacing of the dice
final float DIE_SIZE = X_SPACING*0.8; //width and height of one die
final float X_LEFT_DIE = X_SPACING*0.1; //left side of the leftmost die
final float Y_OFFSET = X_SPACING*0.15; //slight Y offset of the odd-numbered ones
final float Y_POSITION = height-DIE_SIZE-Y_OFFSET; //Y coordinate of most dice
final float PIP_OFFSET = DIE_SIZE/3.5; //Distance from centre to pips, and between pips
final float PIP_DIAM = DIE_SIZE/5; //Diameter of the pips (dots)
//From the constants above, and which die it is, find its top left corner
float dieX = X_LEFT_DIE+position*X_SPACING;
float dieY = Y_POSITION-Y_OFFSET*(position%2);
//1.Draw a red square with a black outline
stroke(0); //Black outline
fill(255,0,0); //Red fill
rect(dieX,dieY,DIE_SIZE,DIE_SIZE);
//2.Draw the pips (dots)
fill(255); //White dots
stroke(255); //White outline
//The centre dot (if the value is odd)
if(1 == value%2)
ellipse(dieX+DIE_SIZE/2,dieY+DIE_SIZE/2,PIP_DIAM,PIP_DIAM);
//The top-left and bottom-right dots (if the value is more than 1)
if(value>1){
ellipse(dieX+DIE_SIZE/2-PIP_OFFSET,
dieY+DIE_SIZE/2+PIP_OFFSET,PIP_DIAM,PIP_DIAM);
ellipse(dieX+DIE_SIZE/2+PIP_OFFSET,
dieY+DIE_SIZE/2-PIP_OFFSET,PIP_DIAM,PIP_DIAM);
}//if
//The bottom-left and top-right dots (if the value is more than 3)
if(value>3){
ellipse(dieX+DIE_SIZE/2+PIP_OFFSET,
dieY+DIE_SIZE/2+PIP_OFFSET,PIP_DIAM,PIP_DIAM);
ellipse(dieX+DIE_SIZE/2-PIP_OFFSET,
dieY+DIE_SIZE/2-PIP_OFFSET,PIP_DIAM,PIP_DIAM);
}//if
//The left and right dots (if the value is 6 or more)
if(value>=6){
ellipse(dieX+DIE_SIZE/2-PIP_OFFSET,
dieY+DIE_SIZE/2,PIP_DIAM,PIP_DIAM);
ellipse(dieX+DIE_SIZE/2+PIP_OFFSET,
dieY+DIE_SIZE/2,PIP_DIAM,PIP_DIAM);
}//if
//The top and bottom centre dots (if the value is 8 or more)
//Normal dice don't have these, but this function allows for
//dice with up to 9 "sides" (values from 1-9).
if(value>=8){
ellipse(dieX+DIE_SIZE/2,
dieY+DIE_SIZE/2-PIP_OFFSET,PIP_DIAM,PIP_DIAM);
ellipse(dieX+DIE_SIZE/2,
dieY+DIE_SIZE/2+PIP_OFFSET,PIP_DIAM,PIP_DIAM);
}//if
}//drawDie
![BRONZE
Frequency and maximum functions
For a lot of the scoring in this game, it is necessary to know the number of times that each number
(1 to 6) appears on the dice, and what the maximum number of times is ("3 of a kind", “4 of a
kind", etc.). Start with the supplied file Lab11BronzeTemplate.pde and add the following two
small functions, in the place indicated. You will also need the two Bronze functions from Lab 10.
1. Write a function int[] freqCount(int[] dice) which will accept an array representing the
numbers on the dice (an array of NUM_DICE values, each from 1 to NUM_SIDES). It should create and
return an array of NUM_SIDES elements indicating how many times each number from 1 to
NUM_SIDES appeared. For example, freqCount (new int[]{1,6,2,1,4}) should return
{2,1,0,1,0,1}. Note that since the indices of an array always start at 0, but dice values start at 1, the
number of 4's would be at index [3].
2. Write a function int maxOfAKind(int[] freqs) which accepts any array of integers, and returns
the biggest one. In terms of the game, if you give it the frequency count from freqCount, it will tell
you how many “of a kind" you have. For example, max0fAKind(new int[]{0,1,3,0,1,1}) should
return 3.
The supplied template will allow you to roll 5 dice, and then it will test the above two functions on the
5 dice obtained, printing the results that they give.
For example, if you rolled the dice 1-4-3-4-4 the program will print
Frequencies of 1..6 are:
1 0 1 3 0 0
(one 1, one 3, three 4's – this is the array freqCount should return)
(this is what max0fAKind should give)
Largest one is 3 of a kind.
You can increase the number of dice (say from 5 to 10) to get a better test. Your functions should both
still work.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe0dd0615-5392-46ed-af9f-2afbe8658b62%2F77f5bc58-92d6-4340-8217-0475e3257699%2Fcmopv8_processed.png&w=3840&q=75)
![18 //------
Bronze
19
20 //Insert all of your Bronze code here
21 int[] DICE_ROLL = new int [NUM_DICE]; //play by rolling 5 dices
22
23 void rollDice() {
for (int a=0; a<NUM_DICE; a++) {
DICE_ROLL[a]= (int)(random ( NUM_SIDES));
}//random (MAX= [0...Max) exclude max 5.999
24
25
26
27 }//rollDice
28
29 void showDiceRoll() {
30
for (int b=0; b<NUM_DICE; b++) {
drawDie (b, DICE_ROLL[b]);
31
32
}
33 }//showDiceRoll](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe0dd0615-5392-46ed-af9f-2afbe8658b62%2F77f5bc58-92d6-4340-8217-0475e3257699%2Fwkxa92g_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

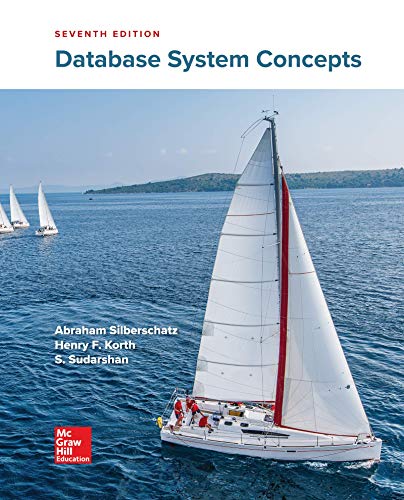
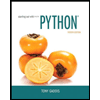
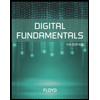
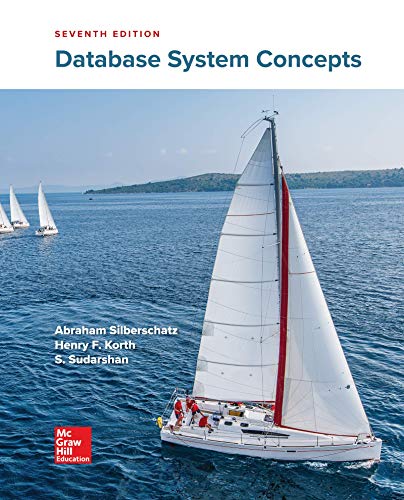
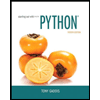
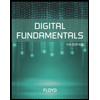
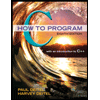
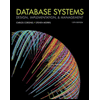
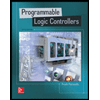