Garbage collection 3. If garbage collection were done just after the final sample output line is printed, explain how the basic mark-and-sweep algorithm would mark heap memory and what it could now sweep up. Since there are multiple instances of each type that is part of this answer, please be sure to uniquely distinguish each object you discuss.
Garbage collection
3. If garbage collection were done just after the final sample output line is printed, explain how the basic mark-and-sweep
// Grades for a student
struct StuGrades {
string name;
int numQuiz;
int* scores;
};
// Read through file to find student with given
// If found, return pointer to new object with name and grades
StuGrades* readStudent(string nm, string filename) {
string nextName, buf;
ifstream in(filename);
while (in >> nextName) {
if (nm == nextName) {
StuGrades* result = new StuGrades;
result->name = nextName;
in >> result->numQuiz;
result->scores = new int[result->numQuiz];
for (int i = 0; i < result->numQuiz; i++) {
in >> result->scores[i];
}
in.close();
return result;
}
else // skip line
getline(in, buf);
}
in.close();
return NULL;
}
int main() {
string more = "y";
string nm;
while (more == "y") {
cout << "Enter name of student to lookup: ";
cin >> nm;
StuGrades* ans = readStudent(nm, "grades.txt");
if (ans != NULL)
cout << ans->name << ' ' << ans->numQuiz << endl;
for (int i = 0; i < ans->numQuiz; i++)
cout << ' ' << ans->scores[i];
cout << endl;
cout << "Keep searching? (y/n) ";
cin >> more;
}
return 0;
}
Here is the sample run:
Enter name of student to lookup: Lee
Lee 2
90 100
Keep searching? (y/n) y
Enter name of student to lookup: Jones
Jones 3
80 90 85
Keep searching? (y/n) y
Enter name of student to lookup: Lee
Lee 2
90 100 ß Q3: Just after this line is output
To help you visualize things, here is a grades.txt file that could give you the above output:
Lee 2 90 100
Jones 3 80 90 85
4. Using sample values with explanations of how the variables in the code are represented in memory, walk through an example of calling the guess() method for the Autoguess struct if it had used a “value receiver” instead of a pointer receiver. The goal is to show why it must be a pointer receiver.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

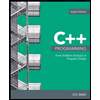
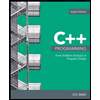