I need a help with some functionalities to enable me implement my code. The Java program uses Genetic Algorithms to recognize a string. Below is the code and a description of the intended purpose as well as a Flow Chart diagram that described the process of how the program should work. The program is intended to work as follows: The user can input the string to guess. The user can enter the number or organisms in the population. The user can enter the number of generations to create. The user can enter the mutation probability. The user can observe the progress of the GA, for example print the best answer in every 10th generation. After the prescribed number of generations, the program should print best/final answer. import java.util.Scanner; public class GATest { public static void main(String[] arg) { boolean repeat = true; while(repeat) { Scanner myScanner = new Scanner(System.in); System.out.print("\nEnter string to guess--»"); String goal = myScanner.nextLine(); System.out.print("Enter number of organisms per generation--»"); int popSize = Integer.parseInt(myScanner.next()); System.out.print("Enter number of generations--»"); int generations = Integer.parseInt(myScanner.next()); System.out.print("Enter mutation probability--»"); double mutateProb = Double.parseDouble(myScanner.next()); System.out.println(); Population aPopulation = new Population(goal, popSize, generations, mutateProb); aPopulation.iterate(); System.out.println("Repeat? y/n"); String answer = myScanner.next(); if (answer.toUpperCase().equals("Y")) repeat = true; else repeat = false; } } } import java.util.Random; public class Organism implements Comparable { String value, goalString; double fitness; int n; Random myRandom = new Random(); public Organism(String goalString) { value=""; this.goalString=goalString; this.n= goalString.length(); for(int i=0; imutateProb) newString = newString+value.charAt(i); else { int j = myRandom.nextInt(27); if (j==26) newString=newString+" "; else { int which = myRandom.nextInt(2); if (which ==0) j=j +65; else j=j+97; newString = newString+(char)j; } } } this.setValue(newString); } } The Organism class Member variables A String or Array of char or an ArrayList of Character. This String represents the Organism’s guess for the unknown String. The number of characters in the Organism should be the same as the number of characters in the unknown String.
I need a help with some functionalities to enable me implement my code. The Java program uses Genetic
The program is intended to work as follows:
- The user can input the string to guess.
- The user can enter the number or organisms in the population.
- The user can enter the number of generations to create.
- The user can enter the mutation probability.
- The user can observe the progress of the GA, for example print the best answer in every 10th generation.
- After the prescribed number of generations, the program should print best/final answer.
import java.util.Scanner;
public class GATest {
public static void main(String[] arg) {
boolean repeat = true;
while(repeat) {
Scanner myScanner = new Scanner(System.in);
System.out.print("\nEnter string to guess--»");
String goal = myScanner.nextLine();
System.out.print("Enter number of organisms per generation--»");
int popSize = Integer.parseInt(myScanner.next());
System.out.print("Enter number of generations--»");
int generations = Integer.parseInt(myScanner.next());
System.out.print("Enter mutation probability--»");
double mutateProb = Double.parseDouble(myScanner.next());
System.out.println();
Population aPopulation = new Population(goal, popSize, generations, mutateProb);
aPopulation.iterate();
System.out.println("Repeat? y/n");
String answer = myScanner.next();
if (answer.toUpperCase().equals("Y"))
repeat = true;
else
repeat = false;
}
}
}
import java.util.Random;
public class Organism implements Comparable<Organism> {
String value, goalString;
double fitness;
int n;
Random myRandom = new Random();
public Organism(String goalString) {
value="";
this.goalString=goalString;
this.n= goalString.length();
for(int i=0; i<n; i++) {
int j = myRandom.nextInt(27);
if(j==26)
value=value+" ";
int which = myRandom.nextInt(2);
if(which==0)
j=j+65;
else
j=j+97;
value=value+ (char)j;
}
}
public Organism(String goalString, String value, int n) {
this.goalString=goalString;
this.value = value;
this.n=n;
}
public Organism() {
}
public String getValue() {
return this.value;
}
public void setValue(String value) {
this.value = value;
}
public String toString() {
return value +" "+ goalString+" "+getFitness(goalString);
}
public int getFitness(String aString) {
int count =0;
for(int i=0; i< this.n; i++)
if(this.value.charAt(i)== aString.charAt(i))
count++;
return count;
}
public int compareTo(Organism other) {
int thisCount, otherCount; thisCount=getFitness(goalString); otherCount=other.getFitness(goalString);
if (thisCount == otherCount)
return 0;
else if (thisCount < otherCount)
return 1;
else
return -1;
}
public Organism[] mate(Organism other) {
Random aRandom = new Random();
int crossOver = aRandom.nextInt(n);
String child1="", child2="";
for (int i=0; i< crossOver; i++) {
child1=child1+this.value.charAt(i);
child2 = child2+other.value.charAt(i);
}
for (int i= crossOver; i<n; i++) {
child1=child1+other.value.charAt(i);
child2=child2+this.value.charAt(i);
}
Organism[] children= new Organism[2];
children[0] = new Organism(goalString, child1,n);
children[1] = new Organism(goalString, child2, n);
return children;
}
public void mutate(double mutateProb) {
String newString="";
for (int i=0; i< n; i++) {
int k = myRandom.nextInt(100);
if (k/100.0 >mutateProb)
newString = newString+value.charAt(i);
else {
int j = myRandom.nextInt(27);
if (j==26)
newString=newString+" ";
else {
int which = myRandom.nextInt(2);
if (which ==0)
j=j +65;
else
j=j+97;
newString = newString+(char)j;
}
}
}
this.setValue(newString);
}
}
The Organism class
Member variables
- A String or Array of char or an ArrayList of Character. This String represents the Organism’s guess for the unknown String. The number of characters in the Organism should be the same as the number of characters in the unknown String.


Trending now
This is a popular solution!
Step by step
Solved in 5 steps

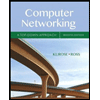
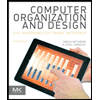
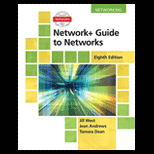
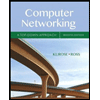
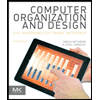
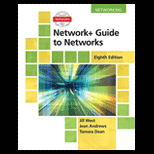
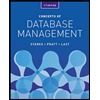
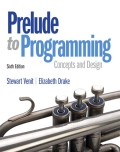
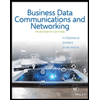