In this project, you will complete a C-class by writing a copy constructor, destructor, and assignment operator. Furthermore, you will write a tester class and a test program and use Valgrind to check that your program is free of memory leaks. Finally, you will submit your project files on GL. If you have submitted programs on GL using shared directories (instead of the submit command), then the submission steps should be familiar.
In this project, you will complete a C-class by writing a copy constructor, destructor, and assignment operator. Furthermore, you will write a tester class and a test program and use Valgrind to check that your program is free of memory leaks. Finally, you will submit your project files on GL. If you have submitted programs on GL using shared directories (instead of the submit command), then the submission steps should be familiar.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:Introduction
In this project, you will complete a C++ class by writing a copy constructor, destructor, and assignment
operator. Furthermore, you will write a tester class and a test program and use Valgrind to check that
your program is free of memory leaks. Finally, you will submit your project files on GL. If you have
submitted programs on GL using shared directories (instead of the submit command), then the
submission steps should be familiar.
In this project we develop an application which holds the information about a digital art piece. There
are two classes in this project.
• The Random class generates data. The implementation of this class is provided. It generates
random int numbers for colors. To create an object of this class we need to specify min and max
values for the constructor. The colors fall between 30 and 37 inclusively.
The Art class generates random colors and stores the color values. You implement the Art class.
An object of the Art class is a digital masterpiece. The class stores the color values for every part
of the masterpiece in a cell of a 2D array. The following figure presents multiple examples of a
masterpiece presentation.
The following figure represents the main data structure in this project. It is a 2d structure.
Assignment
Step 1: Create your working directory
Create a directory in your GL account to contain your project files. For example, cs341/projo.
Step 2: Copy the project files
You can right-click on the file linkes on this page and save-as in your computer. Then, transfer the files
to your GL account using SFTP.
For this project, you are provided with the skeleton .h and.cpp files, a sample driver, and the output of
driver program:
. art.h - The interface for all Random and Art classes.
art.cpp -The skeleton for the Art class implementation.
driver.cpp - The sample driver/test program.
driver.pdf - The output of sample driver/test program.
Step 3: Complete the Art class
The Art class has three data members. The member variable m_masterPiece holds a pointer to an
array of pointers to multiple arrays. The class needs to allocate required memory dynamically. Every
cell in this 2d structure holds an int number which represents a color code. The member variable
m_numRows and m_numColumns define the size of the masterpiece. The variable m_numRows
defines the height and the variable m_numColumns defines the width of the object.
Art:Art()
This is the default constructor. It creates an empty object. An empty object does not hold any memory.
The constructor must initialize all member variables. The empty object has zero height and width.
Art::Art(int rows, int columns)
This is the constructor. It initializes all member variables and it allocates required memory if neccessary.
If the user passes invalid parameters such a a negative size the constructor creates an empty object.
Art: Art
This is the destructor and it deallocates the memory.
void Art:clear()
This function deallocates all memory and reinitializes all member variables to default values. It clears

Transcribed Image Text:the current object to an empty object.
void Art:create(int seed)
This function generates random color code and inserts it into m_masterPiece. To generate random
code the function must create a Random object initialized for generating int numbers. Please note, you
do not modify the Random class. You only use the Random class. The parameter seed is the seed
value to be used by the Random class.
Art:Art(const Art& rhs)
This is the copy constructor. It creates a deep copy of the rhs object. Deep copy means the new object
has its own memory allocated.
const Art& Art:operator=(const Art& rhs)
This is the assignment operator. It creates a deep copy of rhs. Reminder: an assignment operator
needs the protection against self-assignment.
bool Art:appendToRight(const Art& rhs)
This function appends the rhs object to the right of the current object. The append operation only
happens if both objects have the same height or either object is empty. If the operation is successful
the function returns true otherwise it returns false. The function allows for self-append, an object can
be appended to the right of itself. Please note, appending requires reallocation of memory.
bool Art:appendToBottom(const Art& bottom)
This function appends the rhs object to the bottom of the current object. The append operation only
happens if both objects have the same width or either object is empty. If the operation is successful
the function returns true otherwise it returns false. The function allows for self-append, an object can
be appended to the bottom of itself. Please note, appending requires reallocation of memory.
void Art::dumpColors(string pixel)
This function prints the object to the standard output. The implementation of this function is provided.
You do not need to modify this function. This function is for debugging purposes. The parameter pixel
is the character or the string that will be printed. Using a utf-8 character such as "\u2588" can create
interesting effects. If you are using a Windows computer for development you can use ASCII
characters such as "#"since Windows shell does not support utf-8 by default. The File windows.pdf
provides instruction to activate the support of UTF characters.
void Art:dumpValues()
This function prints the color codes to the standard output. The implementation of this function is
provided. You do not need to modify this function. This function is for debugging purposes.
Step 4: Test your code
The sample driver.cpp file will not be accepted as a test file.
.
The name of the test file must be mytest.cpp.
The mytest.cpp file contains the Tester class, and the main function.
. The test cases must be implemented in the Tester class, and must be called in the main function.
. Every test function returns true or false to indicate the pass or fail.
.
A dump() function is provided for debugging purposes, visual inspections will not be accepted as
test cases.
You must write and submit a test program along with the implementation of the Art class. To test your
Art class, you implement your test functions in the Tester class. The Tester class resides in your test file.
You name your test file mytest.cpp. It is strongly recommended that you read the testing guidelines
before writing test cases. A sample test program including Tester class, and sample test functions are
provided in driver.cpp. You add your Tester class, test functions and test cases to mytest.cpp. You must
write a separate function for every test case.
Any program should be adequately tested. Adequate testing includes testing the functionaly for
normal cases, edge cases and error cases. The following list presents some examples:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
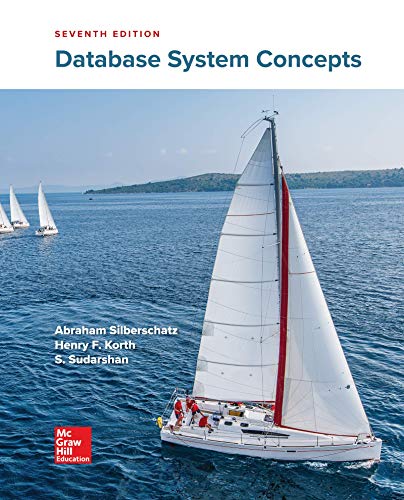
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
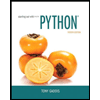
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
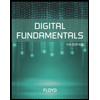
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
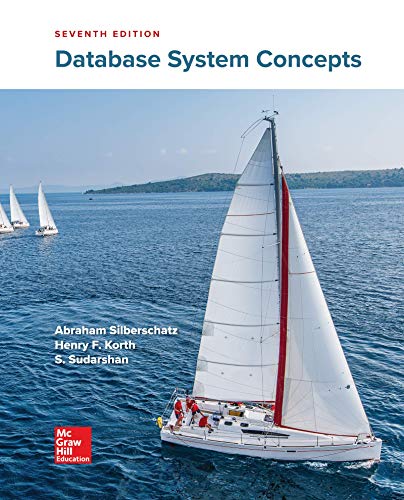
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
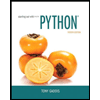
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
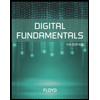
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
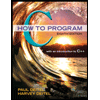
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
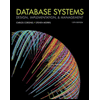
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
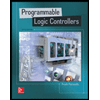
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education