nd concentration is “Data Science”. Print out Alex’s major, gpa, year, and use isHonors method to see if the he is an honors student. Print out Mary’s major, concentration, and years spent in graduate school. Create an object of class Exchange ca
Need help in this question. Must be in java.
the class diagram with four classes student, graduate, undergraduate, and exchange is given. Write the classes as described in the class diagram. The fields and methods for each class is given below.
Fields: major (String)
Student:
gpa (double)
creditHours (int)
Methods:
getGpa: returns gpa
getYear: returns “freshman”, “sophomore”, “junior” or “senior” as determined by earned credit hours:
- Freshman: Less than 32 credit hours
- Sophomore: At least 32 credit hours but less than 64 credit hours
- Junior: At least credit hours 64 but less than 96 credit hours
- Senior: At least 96 credit hours
Graduate:
Fields: degree (String) (“Masters” or “PhD”)
concentration (String)
years (int) (stores no. of years spent in grad school)
Methods:
getYear: returns years (this is an integer, not like the student…)
getConcentration: returns concentration (ex: Data Science, NeuroScience, Bioinformatics,
Undergraduate:
Fields: honors (Boolean)
Methods:
isHonors: returns honors (TRUE or FALSE)
Exchange:
Fields: country (String)
year(string) (start date of the exchange program, stores Fall, Spring or Summer followed by the year e.g., Fall 2020)
Methods:
getYear: returns year
getCountry: returns country
- Write a client class, called studentClient.
- Create an object of class Undergraduate called Alex whose major is CS, gpa is 3.9, credit hours = 54, and honors = TRUE.
- Create an object of class Graduate called Mary whose degree is “Masters”, years = 1, gpa is 3.91, major = “Computer Science” and concentration is “Data Science”.
- Print out Alex’s major, gpa, year, and use isHonors method to see if the he is an honors student.
- Print out Mary’s major, concentration, and years spent in graduate school.
- Create an object of class Exchange called YingShu, for an exchange student with Fall 2020, from Tiawan, and print out their gpa, major, and country.

class student.java
public class Student {
private String major;
private double gpa;
private int creditHours;
//Getters
protected String getMajor() {
return major;
}
protected double getGpa() {
return gpa;
}
protected int getCreditHours() {
return creditHours;
}
protected void setMajor(String newMajor) {
major = newMajor;
}
protected void setGpa(double newGpa) {
gpa = newGpa;
}
protected void setCreditHours(int newCreditHours) {
creditHours = newCreditHours;
}
public Student(String newMajor, double newGpa, int newCreditHours) {
major = newMajor;
gpa = newGpa;
creditHours = newCreditHours;
}
public String getYear() {
if (creditHours < 32) {
return "Freshman";
} else if (creditHours > 32 && creditHours < 64) {
return "Sophomore";
} else if (creditHours > 64 && creditHours < 96) {
return "Junior";
} else if (creditHours > 96) {
return "Senior";
} else {
return "Error 404, Computer Exploding";
}
}
public String toString() {
return "Major: " + major + ", GPA: " + gpa + ", Credit Hours: " + creditHours;
}
}
class StudentClient.java
public class StudentClient {
public static void main(String[] args) {
Undergraduate Maya = new Undergraduate("CS", 3.9, 54);
Maya.setHonors(true);
Graduate John = new Graduate("Computer Science", 4.10, 0);
John.setConcentration("Cybersecurity");
John.setYears(1);
John.setDegree("Masters");
Exchange ExchangeStudent = new Exchange("Chemistry", 3.85, 54);
ExchangeStudent.setYear("Summer 2017");
ExchangeStudent.setCountry("Philippines");
System.out.println("(Maya) Major: " + Maya.getMajor() + ", GPA: " + Maya.getGpa() + ", Year: " + Maya.getYear());
System.out.println("Does Maya have honors? " + Maya.isHonors());
System.out.println();
System.out.println("(John) Concentration: " + John.getConcentration() + ", Years Spent in Grad School: " + John.getYear() + ", Major: " + John.getMajor());
System.out.println();
System.out.println("(Exchange Student) GPA: " + ExchangeStudent.getGpa() + ", Major: " + ExchangeStudent.getMajor() + ", Country: " + ExchangeStudent.getCountry());
System.out.println();
}
}
class Undergraduate.java
public class Undergraduate extends Student {
public Undergraduate(String newMajor, double newGpa, int newCreditHours) {
super(newMajor, newGpa, newCreditHours);
}
private boolean honors;
public boolean isHonors() {
return honors;
}
public boolean getHonors() {
return honors;
}
public void setHonors(boolean newBoolean) {
honors = newBoolean;
}
}
public class Undergraduate extends Student {
public Undergraduate(String newMajor, double newGpa, int newCreditHours) {
super(newMajor, newGpa, newCreditHours);
}
private boolean honors;
public boolean isHonors() {
return honors;
}
public boolean getHonors() {
return honors;
}
public void setHonors(boolean newBoolean) {
honors = newBoolean;
}
}
class Graduate.java
public class Graduate extends Student {
public Graduate(String newMajor, double newGpa, int newCreditHours) {
super(newMajor, newGpa, newCreditHours);
}
private String degree = "masters";
private String concentration;
private int years = 0;
public String getDegree() {
return degree;
}
public String getConcentration() {
return concentration;
}
public String getYear() {
return Integer.toString(years);
}
public void setDegree(String newDegree) {
degree = newDegree;
}
public void setConcentration(String newConcentration) {
concentration = newConcentration;
}
public void setYears(int newYears) {
years = newYears;
}
}
class Exchange.java
public class Exchange extends Undergraduate {
public Exchange(String newMajor, double newGpa, int newCreditHours) {
super(newMajor, newGpa, newCreditHours);
}
private String country;
private String year = "Fall 2019";
public String getYear() {
return year;
}
public String getCountry() {
return country;
}
public void setYear(String newYear) {
year = newYear;
}
public void setCountry(String newCountry) {
country = newCountry;
}
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

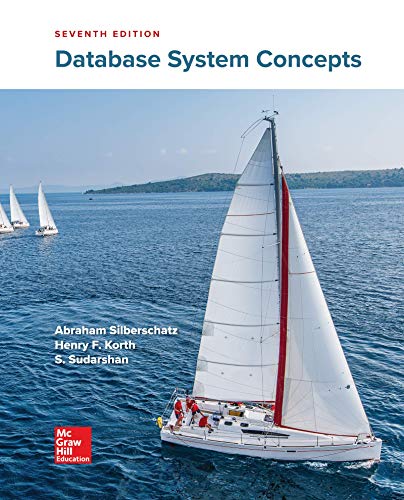
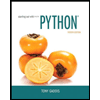
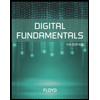
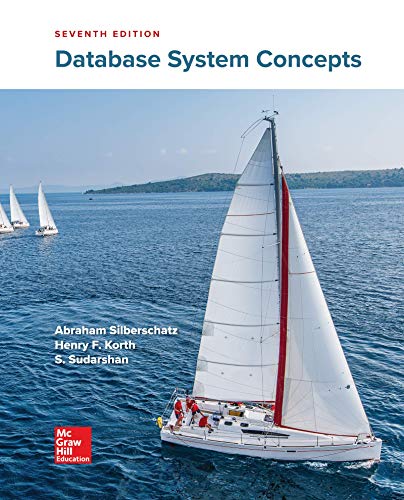
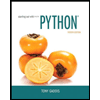
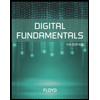
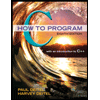
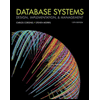
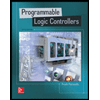