Part 4: Hexadecimal Representation (Base 16) Another commonly used number system in computer science is hexadecimal, which is base 16. That means each digit has 16 possible values and the position of each digit going from right to left represents increasing powers of 16. In order to represent 16 possible values in a single digit, we use letters to represent the numbers greater than 9: A B C D E F 10 11 12 13 14 15 The process for converting a hexadecimal number to decimal is the same as for binary, just using powers of 16. Here is an example converting the number 1B7 to decimal: 1 B 7 256's place 16's place 1's place 16^2 16^1 16^0 (16^2 * 1) + (16^1 * 11) + (16^0 * 7) = (the letter B represents 11 as shown in the table above) (256 * 1) + (16 * 11) + (1 * 7) = 256 + 176 + 7 = 439 So the number 439 in hexadecimal is 1B7. You can also convert decimal to hexadecimal using the same division method as binary, except by dividing by 16. Remember to convert the remainders over 9 into the corresponding letters. To differentiate between numbers in different number systems, you will sometimes see the numbers with a radix letter written after them. We use "d" for decimal, "b" for binary, and "h" for hexadecimal. This way we don't get confused about which base system a number is using. Number with radix Number system Value when converted to decimal 100d decimal (base 10) 100 100b binary (base 2) 4 100h hexadecimal (base 16) 256 The reason we use hexadecimal is because it can represent binary numbers in a fraction of the written space. A byte of binary would normally take up 8 digits, but that same number can be written using just 2 hexadecimal digits: 11010100 = D4 Converting between the two is also very easy - each set of 4 binary bits directly corresponds to a single digit of hexadecimal. You can take each set of 4 binary bits, convert them individually, then stick them back together. This also works in reverse. Binary 1101 0100 To decimal 13 4 To Hexadecimal D 4 Exercise 8: What is the hexadecimal number FA in decimal? Exercise 9: What is the hexadecimal number 3B8F in binary? The result will be 16 digits, since each digit in hexadecimal corresponds to 4 digits in binary. Part 5: Character Sets We've now covered how to represent numbers in computer memory. How do we represent letters and other characters, such as punctuation? The answer is thankfully very simple. We give a number to each symbol that is possible to type (including capital letters, lowercase letters, punctuation, spaces, underscores, slashes, number digits, math symbols, and more) and store text as those encoded numbers. There are standardized tables called "character sets", which keep track of the codes given to each symbol. One of the most common character sets is called ASCII, which is also covered in the discussion assignment for this week. Other character sets include ANSI and Unicode. In this class we will exclusively use ASCII. One advantage of ASCII is that every character can be represented using just a single byte. An ASCII table has been provided in this module for your reference, and you can find one easily at any time by Googling "ascii table". Use the ASCII table to answer the following exercises. Exercise 10: How is the letter "d" represented in binary? (Hint: find "d" on the ASCII table and look at its decimal or hexadecimal code, then convert that to binary. Note that uppercase D and lowercase d have different codes!). Exercise 11: Convert the following binary to text by using the process from the previous exercise in reverse. Each set of 8 bits represents a single character. Remember that capitalization matters! 01001110 01101001 01100011 01100101
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Part 4: Hexadecimal Representation (Base 16)
Another commonly used number system in computer science is hexadecimal, which is base 16. That means each digit has 16 possible values and the position of each digit going from right to left represents increasing powers of 16. In order to represent 16 possible values in a single digit, we use letters to represent the numbers greater than 9:
A | B | C | D | E | F |
10 | 11 | 12 | 13 | 14 | 15 |
The process for converting a hexadecimal number to decimal is the same as for binary, just using powers of 16. Here is an example converting the number 1B7 to decimal:
1 | B | 7 |
256's place | 16's place | 1's place |
16^2 | 16^1 | 16^0 |
(16^2 * 1) + (16^1 * 11) + (16^0 * 7) = (the letter B represents 11 as shown in the table above)
(256 * 1) + (16 * 11) + (1 * 7) =
256 + 176 + 7 =
439
So the number 439 in hexadecimal is 1B7.
You can also convert decimal to hexadecimal using the same division method as binary, except by dividing by 16. Remember to convert the remainders over 9 into the corresponding letters.
To differentiate between numbers in different number systems, you will sometimes see the numbers with a radix letter written after them. We use "d" for decimal, "b" for binary, and "h" for hexadecimal. This way we don't get confused about which base system a number is using.
Number with radix | Number system | Value when converted to decimal |
100d | decimal (base 10) | 100 |
100b | binary (base 2) | 4 |
100h | hexadecimal (base 16) | 256 |
The reason we use hexadecimal is because it can represent binary numbers in a fraction of the written space. A byte of binary would normally take up 8 digits, but that same number can be written using just 2 hexadecimal digits:
11010100 = D4
Converting between the two is also very easy - each set of 4 binary bits directly corresponds to a single digit of hexadecimal. You can take each set of 4 binary bits, convert them individually, then stick them back together. This also works in reverse.
Binary | 1101 | 0100 |
To decimal | 13 | 4 |
To Hexadecimal | D | 4 |
Exercise 8: What is the hexadecimal number FA in decimal?
Exercise 9: What is the hexadecimal number 3B8F in binary? The result will be 16 digits, since each digit in hexadecimal corresponds to 4 digits in binary.
Part 5: Character Sets
We've now covered how to represent numbers in computer memory. How do we represent letters and other characters, such as punctuation?
The answer is thankfully very simple. We give a number to each symbol that is possible to type (including capital letters, lowercase letters, punctuation, spaces, underscores, slashes, number digits, math symbols, and more) and store text as those encoded numbers. There are standardized tables called "character sets", which keep track of the codes given to each symbol. One of the most common character sets is called ASCII, which is also covered in the discussion assignment for this week. Other character sets include ANSI and Unicode.
In this class we will exclusively use ASCII. One advantage of ASCII is that every character can be represented using just a single byte. An ASCII table has been provided in this module for your reference, and you can find one easily at any time by Googling "ascii table". Use the ASCII table to answer the following exercises.
Exercise 10: How is the letter "d" represented in binary? (Hint: find "d" on the ASCII table and look at its decimal or hexadecimal code, then convert that to binary. Note that uppercase D and lowercase d have different codes!).
Exercise 11: Convert the following binary to text by using the process from the previous exercise in reverse. Each set of 8 bits represents a single character. Remember that capitalization matters!
01001110 01101001 01100011 01100101

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

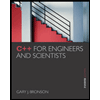
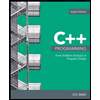
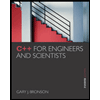
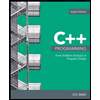
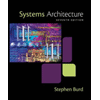