Please help me with this Please fix the full code: this code is supposed to work in a web browser (Client_side.js) const url = "http://localhost:3000/document"; let undoStack = [];let redoStack = []; function sendDataToServer(data) { fetch(url, { method: 'POST', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify({ content: data }) }) .then(response => response.json()) .then(data => console.log('Success:', data)) .catch((error) => console.error('Error:', error));} function formatText(command) { document.execCommand(command, false, null);} function changeColor() { const color = prompt("Enter text color code:"); document.execCommand("foreColor", false, color);} function changeFont() { const font = prompt("Enter font:"); document.execCommand("fontName", false, font);} function changeFontSize() { const size = prompt("Enter font size:"); document.execCommand("fontSize", false, size);} function clearText() { document.getElementById("editor").innerHTML = "";} function toUpperCase() { const selectedText = window.getSelection().toString(); document.execCommand("insertText", false, selectedText.toUpperCase());} function toLowerCase() { const selectedText = window.getSelection().toString(); document.execCommand("insertText", false, selectedText.toLowerCase());} function highlightText() { const color = prompt("Enter highlight color code:"); document.execCommand("hiliteColor", false, color);} function createTable() { const rows = prompt("Enter the number of rows:"); const cols = prompt("Enter the number of columns:"); let tableHTML = "<table border='1'>"; for (let i = 0; i < rows; i++) { tableHTML += "<tr>"; for (let j = 0; j < cols; j++) { tableHTML += "<td>Cell</td>"; } tableHTML += "</tr>"; } tableHTML += "</table>"; document.execCommand("insertHTML", false, tableHTML);} function increaseImageSize() { const selectedImage = document.querySelector('img.resizable'); if (selectedImage) { const currentWidth = selectedImage.width; selectedImage.style.width = (currentWidth * 1.2) + 'px'; }} function decreaseImageSize() { const selectedImage = document.querySelector('img.resizable'); if (selectedImage) { const currentWidth = selectedImage.width; selectedImage.style.width = (currentWidth * 0.8) + 'px'; }} function insertImage() { const imageUrl = prompt("Enter the image URL:"); if (imageUrl) { const imgHTML = "<img src='" + imageUrl + "' alt='User Image' class='resizable'>"; document.execCommand("insertHTML", false, imgHTML); }} function undo() { if (undoStack.length > 0) { redoStack.push(document.getElementById("editor").innerHTML); document.getElementById("editor").innerHTML = undoStack.pop(); }} function redo() { if (redoStack.length > 0) { undoStack.push(document.getElementById("editor").innerHTML); document.getElementById("editor").innerHTML = redoStack.pop(); }} function autoSave() { const currentContent = document.getElementById("editor").innerHTML; undoStack.push(currentContent); if (undoStack.length > 10) { undoStack.shift(); } // Implement autosave functionality here (e.g., send currentContent to server for saving)} function updateTextInfo() { const editorContent = document.getElementById("editor").innerText; const wordCount = editorContent.trim().split(/\s+/).filter(word => word.length > 0).length; const characterCount = editorContent.replace(/[\n\s]+/g, '').length; const sentenceCount = editorContent.split(/[.!?]+/).filter(sentence => sentence.length > 0).length; const paragraphCount = editorContent.split('\n\n').filter(para => para.trim() !== '').length; document.getElementById("wordCount").innerText = "Word Count: " + wordCount; document.getElementById("charCount").innerText = "Character Count: " + characterCount; document.getElementById("sentenceCount").innerText = "Sentence Count: " + sentenceCount; document.getElementById("paraCount").innerText = "Paragraph Count: " + paragraphCount;});
Please help me with this
Please fix the full code: this code is supposed to work in a web browser (Client_side.js)
const url = "http://localhost:3000/document";
let undoStack = [];
let redoStack = [];
function sendDataToServer(data) {
fetch(url, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ content: data })
})
.then(response => response.json())
.then(data => console.log('Success:', data))
.catch((error) => console.error('Error:', error));
}
function formatText(command) {
document.execCommand(command, false, null);
}
function changeColor() {
const color = prompt("Enter text color code:");
document.execCommand("foreColor", false, color);
}
function changeFont() {
const font = prompt("Enter font:");
document.execCommand("fontName", false, font);
}
function changeFontSize() {
const size = prompt("Enter font size:");
document.execCommand("fontSize", false, size);
}
function clearText() {
document.getElementById("editor").innerHTML = "";
}
function toUpperCase() {
const selectedText = window.getSelection().toString();
document.execCommand("insertText", false, selectedText.toUpperCase());
}
function toLowerCase() {
const selectedText = window.getSelection().toString();
document.execCommand("insertText", false, selectedText.toLowerCase());
}
function highlightText() {
const color = prompt("Enter highlight color code:");
document.execCommand("hiliteColor", false, color);
}
function createTable() {
const rows = prompt("Enter the number of rows:");
const cols = prompt("Enter the number of columns:");
let tableHTML = "<table border='1'>";
for (let i = 0; i < rows; i++) {
tableHTML += "<tr>";
for (let j = 0; j < cols; j++) {
tableHTML += "<td>Cell</td>";
}
tableHTML += "</tr>";
}
tableHTML += "</table>";
document.execCommand("insertHTML", false, tableHTML);
}
function increaseImageSize() {
const selectedImage = document.querySelector('img.resizable');
if (selectedImage) {
const currentWidth = selectedImage.width;
selectedImage.style.width = (currentWidth * 1.2) + 'px';
}
}
function decreaseImageSize() {
const selectedImage = document.querySelector('img.resizable');
if (selectedImage) {
const currentWidth = selectedImage.width;
selectedImage.style.width = (currentWidth * 0.8) + 'px';
}
}
function insertImage() {
const imageUrl = prompt("Enter the image URL:");
if (imageUrl) {
const imgHTML = "<img src='" + imageUrl + "' alt='User Image' class='resizable'>";
document.execCommand("insertHTML", false, imgHTML);
}
}
function undo() {
if (undoStack.length > 0) {
redoStack.push(document.getElementById("editor").innerHTML);
document.getElementById("editor").innerHTML = undoStack.pop();
}
}
function redo() {
if (redoStack.length > 0) {
undoStack.push(document.getElementById("editor").innerHTML);
document.getElementById("editor").innerHTML = redoStack.pop();
}
}
function autoSave() {
const currentContent = document.getElementById("editor").innerHTML;
undoStack.push(currentContent);
if (undoStack.length > 10) {
undoStack.shift();
}
// Implement autosave functionality here (e.g., send currentContent to server for saving)
}
function updateTextInfo() {
const editorContent = document.getElementById("editor").innerText;
const wordCount = editorContent.trim().split(/\s+/).filter(word => word.length > 0).length;
const characterCount = editorContent.replace(/[\n\s]+/g, '').length;
const sentenceCount = editorContent.split(/[.!?]+/).filter(sentence => sentence.length > 0).length;
const paragraphCount = editorContent.split('\n\n').filter(para => para.trim() !== '').length;
document.getElementById("wordCount").innerText = "Word Count: " + wordCount;
document.getElementById("charCount").innerText = "Character Count: " + characterCount;
document.getElementById("sentenceCount").innerText = "Sentence Count: " + sentenceCount;
document.getElementById("paraCount").innerText = "Paragraph Count: " + paragraphCount;
});

Step by step
Solved in 2 steps

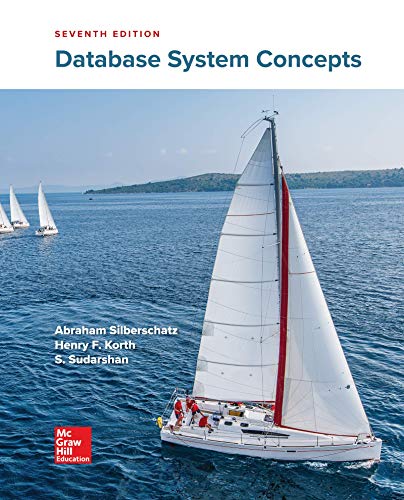
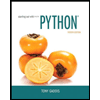
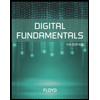
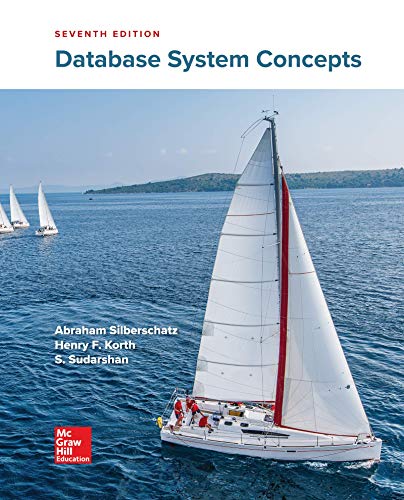
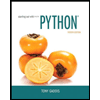
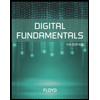
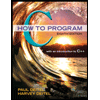
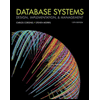
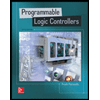