ssume you have a stack implemented with single-linked nodes, that looks like this: Grapefruit -> Peach -> Lime -> Apple -> Banana -> Pineapple 'top' is a reference variable that points to the node containing Grapefruit, and 'count' is an nt that holds the count of nodes (currently 6). If you want to do pop operation, what is the pseudocode to achieve this? O create a String called temp
ssume you have a stack implemented with single-linked nodes, that looks like this: Grapefruit -> Peach -> Lime -> Apple -> Banana -> Pineapple 'top' is a reference variable that points to the node containing Grapefruit, and 'count' is an nt that holds the count of nodes (currently 6). If you want to do pop operation, what is the pseudocode to achieve this? O create a String called temp
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 21SA
Related questions
Question

Transcribed Image Text:Assume you have a stack implemented with single-linked nodes, that looks like this:
Grapefruit -> Peach -> Lime -> Apple -> Banana -> Pineapple
'top' is a reference variable that points to the node containing Grapefruit, and 'count' is an internal
int that holds the count of nodes (currently 6).
If you want to do pop operation, what is the pseudocode to achieve this?
create a String called temp
set temp's pointer to be the same as top's element pointer
set top's pointer to temp
decrement count
return temp
O create a String called temp
set temp's pointer to be the same as top's next pointer
set top's pointer to be the same as top's element pointer
decrement count
return temp

Transcribed Image Text:create single linked node called temp
set temp's element pointer to Peach
set top to point at temp
decrement count
return Grapefruit
create a String called temp
set temp's pointer to be the same as top's element pointer
set top's pointer to be the same as top's next pointer
decrement count
return temp
O create a String called temp
set temp's pointer to be the same as top's element pointer
set top's next pointer to be the same as top's next's next pointer
decrement count
return temp
Expert Solution

Step 1
As we know A singly linked list is a data structure that consists of a sequence of nodes, where each node contains an element and a reference (or "pointer") to the next node in the sequence. The first node in the list is referred to as the "head" node, and the last node in the list doesn't have a reference to another node, indicating the end of the list
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
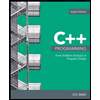
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
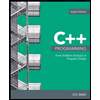
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning