The following functions are all supposed to count how many times a certain base (represented as a character variable in Python) appears in a dna sequence (represented as a string variable in Python): def count1(dna, base): i = 0 for c in dna: if c == base: i += 1 return i def count2(dna, base): i = 0 for j in range(len(dna)): if dna[j] == base: i += 1 return i def count3(dna, base): match = [c == base for c in dna] return sum(match) def count4(dna, base): return dna.count(base) def count5(dna, base): return len([i for i in range(len(dna)) if dna[i] == base]) def count6(dna,base): return sum(c == base for c in dna) ======================================================================================= Which of them is correct? count 3, and count 6 only count 1, count 3, and count 4 only All of them are correct. count 1, count 3, count 4, and count 6 only
The following functions are all supposed to count how many times a certain base (represented as a character variable in Python) appears in a dna sequence (represented as a string variable in Python): def count1(dna, base): i = 0 for c in dna: if c == base: i += 1 return i def count2(dna, base): i = 0 for j in range(len(dna)): if dna[j] == base: i += 1 return i def count3(dna, base): match = [c == base for c in dna] return sum(match) def count4(dna, base): return dna.count(base) def count5(dna, base): return len([i for i in range(len(dna)) if dna[i] == base]) def count6(dna,base): return sum(c == base for c in dna) ======================================================================================= Which of them is correct? count 3, and count 6 only count 1, count 3, and count 4 only All of them are correct. count 1, count 3, count 4, and count 6 only
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter6: Modularity Using Functions
Section6.4: A Case Study: Rectangular To Polar Coordinate Conversion
Problem 9E: (Numerical) Write a program that tests the effectiveness of the rand() library function. Start by...
Related questions
Question
The following functions are all supposed to count how many times a certain base (represented as a character variable in Python) appears in a dna sequence (represented as a string variable in Python):
- def count1(dna, base):
- i = 0
- for c in dna:
- if c == base:
- i += 1
- return i
- def count2(dna, base):
- i = 0
- for j in range(len(dna)):
- if dna[j] == base:
- i += 1
- return i
- def count3(dna, base):
- match = [c == base for c in dna]
- return sum(match)
- def count4(dna, base):
- return dna.count(base)
- def count5(dna, base):
- return len([i for i in range(len(dna)) if dna[i] == base])
- def count6(dna,base):
- return sum(c == base for c in dna)
=======================================================================================
Which of them is correct?
- count 3, and count 6 only
- count 1, count 3, and count 4 only
- All of them are correct.
- count 1, count 3, count 4, and count 6 only
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
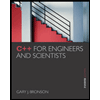
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
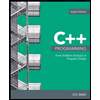
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
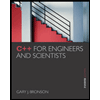
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
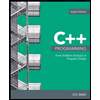
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning