This is the first exercise for the TODO comments in the main method. Complete the actions described in these comment lines in the main method: // TODO: Add a public static constant called PRIMARY_NAME // to the Student class. Set the name of the Primary to // a real University. In other words: Add a public static final String variable called PRIMARY_NAME to the fields of the Student class. Initialise the variable in the same line it is declared (not in the constructor) to the name of a real Primary. Add code to the Main class in this project to access the PRIMARY_NAME field and print it out. Remember that, because the field is public, it can be accessed directly from another class without having to provide a getter method for it. In the lecture discussing static fields and methods, we showed that the nextID field in the Student class is used to store the value of the next student ID to be allocated as each Student is created.This and the following exercises will confirm this behaviour. Complete the actions described in these comment lines in the main method: // Get the number of the next Student id to be allocated // and store it in a local variable. // TODO: Your code here to set nextID. int nextID; In other words, set the nextID local variable in the main method by calling the getter for the nextID field in the Student class. Remember that the getter is a static method, so it must be called on the Student class name rather than on a Student object. Complete the actions described in these comment lines in the main method: // TODO: Your code here to set studentID to the ID // of aStudent. int studentID; In other words, set the studentID local variable by calling the ID getter method on the aStudent Student object. Complete the actions described in these comment lines in the main method: // TODO: Write an if-statement to check that the student just // created has the same ID as that stored in nextID. // Print OK if they have the correct ID. // Print Error if they do not have the correct ID. If other words, compare the values of the nextID and studentID local variables in the main method to make sure they are the same. Print OK if they are or Error if they are not. Complete the actions described in these comment lines in the main method: // TODO: Repeat the above steps to: // + get the nextID to be allocated. // + create a second Student object. // + get the ID of the second Student object. // + check and report whether the IDs match. In other words, get the nextID again from the Student class and store it in the nextID local variable in the main method. Create a second Student object and store it in the aStudent variable. Get the ID of aStudent and store it in the studentID local variable. Compare the values of nextID and StudentID and print either OK or Error.
This is the first exercise for the TODO comments in the main method. Complete the actions described in these comment lines in the main method:
// TODO: Add a public static constant called PRIMARY_NAME
// to the Student class. Set the name of the Primary to
// a real University. In other words: Add a public static final String variable called PRIMARY_NAME to the fields of the Student class. Initialise the variable in the same line it is declared (not in the constructor) to the name of a real Primary. Add code to the Main class in this project to access the PRIMARY_NAME field and print it out. Remember that, because the field is public, it can be accessed directly from another class without having to provide a getter method for it. In the lecture discussing static fields and methods, we showed that the nextID field in the Student class is used to store the value of the next student ID to be allocated as each Student is created.This and the following exercises will confirm this behaviour. Complete the actions described in these comment lines in the main method:
// Get the number of the next Student id to be allocated
// and store it in a local variable.
// TODO: Your code here to set nextID.
int nextID; In other words, set the nextID local variable in the main method by calling the getter for the nextID field in the Student class. Remember that the getter is a static method, so it must be called on the Student class name rather than on a Student object. Complete the actions described in these comment lines in the main method:
// TODO: Your code here to set studentID to the ID
// of aStudent.
int studentID; In other words, set the studentID local variable by calling the ID getter method on the aStudent Student object. Complete the actions described in these comment lines in the main method:
// TODO: Write an if-statement to check that the student just
// created has the same ID as that stored in nextID.
// Print OK if they have the correct ID.
// Print Error if they do not have the correct ID. If other words, compare the values of the nextID and studentID local variables in the main method to make sure they are the same. Print OK if they are or Error if they are not. Complete the actions described in these comment lines in the main method:
// TODO: Repeat the above steps to:
// + get the nextID to be allocated.
// + create a second Student object.
// + get the ID of the second Student object.
// + check and report whether the IDs match. In other words, get the nextID again from the Student class and store it in the nextID local variable in the main method. Create a second Student object and store it in the aStudent variable. Get the ID of aStudent and store it in the studentID local variable. Compare the values of nextID and StudentID and print either OK or Error.

Step by step
Solved in 3 steps with 1 images

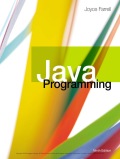
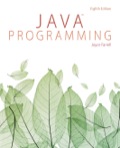
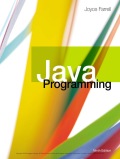
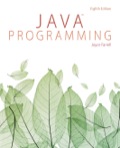
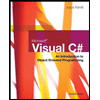