Write classes that could be used in a system that manages vehicles in a public transportation system. You will write classes to represent a TransitVehicle, Bus, and SubwayCar. The information about the classes is below. Step 1: Plan Your Class Design Before you start to code, decide how to design your classes. Which class should be the parent? the child? What variables or methods belong in each class? Step 2: Write the Core Class Components Write the three classes. Each class must have all of the following. Important: a class can have a method directly (meaning written into the code) or indirectly (meaning inherited from a parent). Either of these counts to meet the requirement. instance data variables transit vehicles are described by an id that contains letters and numbers and a description of the route buses are described by an id, route, and the number of miles per gallon (without decimals) subway cars are described by an id, route, and whether or not the car goes underground a constructor that sets all of the instance data variables using parameters getter and setter methods in your setter for the number of miles per gallon, use validity checking to make sure the parameter is valid before assigning it to the instance data variable a toString method formatting of the text representation is provided below in the expected output include the route and id for all vehicles for buses, also include the word "Bus" and the miles per gallon for subway cars, also include the words "Subway" and add a notation if the car goes underground Step 3: Class Specific Methods Write three class specific methods. Decide which class each method belongs in. If a method is in a parent class, decide if it also needs to be overridden in the child class. printSchedule all transit vehicles display their route and id (no actual schedule needs to be shown) repair all transit vehicles display a message that they need repair; the message includes their id for subway cars that go underground, the message also includes a note that the car must be removed from the tunnel fuel buses display a message that they are being given fuel; the message includes the bus id Step 4: Write a Driver Program Write code in a main method to demonstrate your classes. In main: Create a list of transit vehicles using the data below. Iterate the list and print the text representation of each vehicle. Iterate the list and print the schedule for each vehicle. Iterate the list and repair each vehicle. The expected output is shown below. Design and Additional Coding Requirements code should compile the inheritance relationship and method placement should be a logical fit for the classes follow best practices related to inheritance, including letting a parent class be in charge of parent functionality (e.g., by using super(...) and super.method(...)) reduce duplicated code (consider how to replace repeated code with a method or through inheritance) follow Java naming conventions for classes (upper camel case) and for variables and methods (lower camel case with no underscores) choose the best loops and conditional structures for a task use constants instead of hard-coded values when possible to improve clarity and readability and to make code easier to maintain Transit Vehicle Data Bus: route = "Fulton St 5", id = "F12", bus gets 4 miles to the gallon Bus: route = "Geary St 38", id = "G43", bus gets 3 miles to the gallon Bus: route = "Geary St 38L", id = "G92", bus gets 7 miles to the gallon Subway Car: route = "Judah St N", id = "J19", does not go underground Subway Car: route = "Taraval L", id = "T25", goes underground Expected Output Here is what the output of running your driver should be. Note that the spacing does not have to be exact. Route: Fulton St 5 ID: F12 Type: Bus Miles Per Gallon: 4 Route: Geary St 38 ID: G43 Type: Bus Miles Per Gallon: 3 Route: Geary St 38L ID: G92 Type: Bus Miles Per Gallon: 7 Route: Judah St N ID: J19 Type: Subway Route: Taraval L ID: T25 Type: Subway (Underground) Printing Fulton St 5 schedule for vehicle F12 Printing Geary St 38 schedule for vehicle G43 Printing Geary St 38L schedule for vehicle G92 Printing Judah St N schedule for vehicle J19 Printing Taraval L schedule for vehicle T25 Repairs needed for vehicle F12 Repairs needed for vehicle G43 Repairs needed for vehicle G92 Repairs needed for vehicle J19 Repairs needed for vehicle T25 ***Must remove car from tunnel.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Write classes that could be used in a system that manages vehicles in a public transportation system.
You will write classes to represent a TransitVehicle, Bus, and SubwayCar. The information about the classes is below.
Step 1: Plan Your Class Design
Before you start to code, decide how to design your classes. Which class should be the parent? the child? What variables or methods belong in each class?
Step 2: Write the Core Class Components
Write the three classes. Each class must have all of the following.
Important: a class can have a method directly (meaning written into the code) or indirectly (meaning inherited from a parent). Either of these counts to meet the requirement.
- instance data variables
- transit vehicles are described by an id that contains letters and numbers and a description of the route
- buses are described by an id, route, and the number of miles per gallon (without decimals)
- subway cars are described by an id, route, and whether or not the car goes underground
- a constructor that sets all of the instance data variables using parameters
- getter and setter methods
- in your setter for the number of miles per gallon, use validity checking to make sure the parameter is valid before assigning it to the instance data variable
- a toString method
- formatting of the text representation is provided below in the expected output
- include the route and id for all vehicles
- for buses, also include the word "Bus" and the miles per gallon
- for subway cars, also include the words "Subway" and add a notation if the car goes underground
Step 3: Class Specific Methods
Write three class specific methods. Decide which class each method belongs in. If a method is in a parent class, decide if it also needs to be overridden in the child class.
- printSchedule
- all transit vehicles display their route and id (no actual schedule needs to be shown)
- repair
- all transit vehicles display a message that they need repair; the message includes their id
- for subway cars that go underground, the message also includes a note that the car must be removed from the tunnel
- fuel
- buses display a message that they are being given fuel; the message includes the bus id
Step 4: Write a Driver Program
Write code in a main method to demonstrate your classes. In main:
- Create a list of transit vehicles using the data below.
- Iterate the list and print the text representation of each vehicle.
- Iterate the list and print the schedule for each vehicle.
- Iterate the list and repair each vehicle.
The expected output is shown below.
Design and Additional Coding Requirements
- code should compile
- the inheritance relationship and method placement should be a logical fit for the classes
- follow best practices related to inheritance, including letting a parent class be in charge of parent functionality (e.g., by using super(...) and super.method(...))
- reduce duplicated code (consider how to replace repeated code with a method or through inheritance)
- follow Java naming conventions for classes (upper camel case) and for variables and methods (lower camel case with no underscores)
- choose the best loops and conditional structures for a task
- use constants instead of hard-coded values when possible to improve clarity and readability and to make code easier to maintain
Transit Vehicle Data
- Bus: route = "Fulton St 5", id = "F12", bus gets 4 miles to the gallon
- Bus: route = "Geary St 38", id = "G43", bus gets 3 miles to the gallon
- Bus: route = "Geary St 38L", id = "G92", bus gets 7 miles to the gallon
- Subway Car: route = "Judah St N", id = "J19", does not go underground
- Subway Car: route = "Taraval L", id = "T25", goes underground
Expected Output
Here is what the output of running your driver should be. Note that the spacing does not have to be exact.
Route: Fulton St 5 ID: F12 Type: Bus Miles Per Gallon: 4
Route: Geary St 38 ID: G43 Type: Bus Miles Per Gallon: 3
Route: Geary St 38L ID: G92 Type: Bus Miles Per Gallon: 7
Route: Judah St N ID: J19 Type: Subway
Route: Taraval L ID: T25 Type: Subway (Underground)
Printing Fulton St 5 schedule for vehicle F12
Printing Geary St 38 schedule for vehicle G43
Printing Geary St 38L schedule for vehicle G92
Printing Judah St N schedule for vehicle J19
Printing Taraval L schedule for vehicle T25
Repairs needed for vehicle F12
Repairs needed for vehicle G43
Repairs needed for vehicle G92
Repairs needed for vehicle J19
Repairs needed for vehicle T25
***Must remove car from tunnel.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 7 images

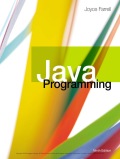
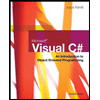
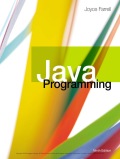
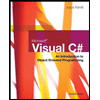