You are required to implement a shopping cart module for an online shopping mall. From the available items a user will select the item to purchase and add this into his shopping cart. Before the checkout customer can remove any item from the cart at any time. A single item in the cart contains the following details: class Item { private: int itemID; string itemName; double price; double purchasedQuantity; double totalPrice; public: //constructors and getter setters }; For the implementation of the required module you have to meet the following requirements: 1- Customer can add any item to the cart in desired quantity. Remember if customer added the same item in the cart again, your implementation will not add this as a new item it will check if the item already exists than it will just add the new quantity in the previous and update other data. 2- Customer can remove item or change the quantity at any time. There are two possibilities: i. Customer wants to remove the item form the cart ii. Customer wants to change the quantity of item in the cart 3- Customer can also view his cart at any time. You have to provide two features for the user in order to view his cart: i. View cart: it will display all the items that customer selected to purchase. ii. View cart by items: Display one item and facilitate user to navigate through next or previous item in the cart till the user wants to quit the view. 4- Customer can calculate his bill. Bill will be calculated according to the item price and the number of items he wants to purchase. 5- Bill should be displayed in user friendly manner to the customer. You have to provide implementation of following methods for the Cart class. addToCart(int itemID,string itemName,double price, int purchasedQuantity); addExistingToCart(int itemID, int quantityToAdd); removeFromCart(item itemToRemove); void changeQuantity : (int itemID, int newQuantity); calculateBill(); printBill(); viewCartByItem(); viewCart(); Instruction: Use double linked list in c++ to implement the above scenario
You are required to implement a shopping cart module for an online shopping mall. From the available items a
user will select the item to purchase and add this into his shopping cart. Before the checkout customer can
remove any item from the cart at any time.
A single item in the cart contains the following details:
class Item
{
private:
int itemID;
string itemName;
double price;
double purchasedQuantity;
double totalPrice;
public:
//constructors and getter setters
};
For the implementation of the required module you have to meet the following requirements:
1- Customer can add any item to the cart in desired quantity.
Remember if customer added the same item in the cart again, your implementation will not add this as a new
item it will check if the item already exists than it will just add the new quantity in the previous and update
other data.
2- Customer can remove item or change the quantity at any time.
There are two possibilities:
i. Customer wants to remove the item form the cart
ii. Customer wants to change the quantity of item in the cart
3- Customer can also view his cart at any time. You have to provide two features for the user in order
to view his cart:
i. View cart: it will display all the items that customer selected to purchase.
ii. View cart by items: Display one item and facilitate user to navigate through next or
previous item in the cart till the user wants to quit the view.
4- Customer can calculate his bill. Bill will be calculated according to the item price and the number
of items he wants to purchase.
5- Bill should be displayed in user friendly manner to the customer.
You have to provide implementation of following methods for the Cart class.
addToCart(int itemID,string itemName,double price, int purchasedQuantity);
addExistingToCart(int itemID, int quantityToAdd);
removeFromCart(item itemToRemove);
void changeQuantity : (int itemID, int newQuantity);
calculateBill();
printBill();
viewCartByItem();
viewCart();
Instruction: Use double linked list in c++ to implement the above scenario

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

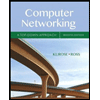
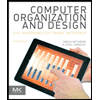
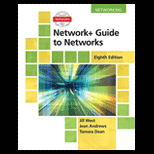
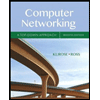
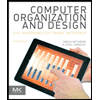
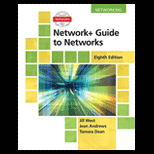
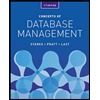
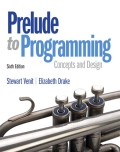
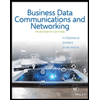