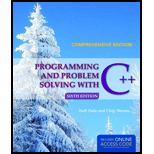
Concept explainers
To input the user's name and the Social Security Number and print the details in different formats.

Explanation of Solution
Program:
#include <iostream> usingnamespace std; intmain() { stringfname, lname; // string declared for the first name and the last name intssn; // integer declared for the Social Security Number cout<<"Enter your first name:\n"; cin>>fname; cout<<"Enter your last name:\n"; cin>>lname; cout<<"Enter your Social Security Number:\n"; cin>>ssn; cout<<"Your details are:\n"; // details will be printed in different formats. cout<<ssn<<", "<<fname<<", "<<lname<<endl; cout<<ssn<<", "<<lname<<", "<<fname<<endl; cout<<lname<<", "<<fname<<", "<<ssn<<endl; return 0; }
Explanation:
An 'iostream' is a header file or a pre-defined file in which cin and cout is defined.
To use the header files in the program, keyword include is written before the header file name surrounded with angular brackets.
The include keyword is always written after the '#', which is known as a preprocessor.
A preprocessor means something is being processed before the program. A preprocessor shows that the header files are processed before the program.
A 'using' is a keyword used to specify namespace or file is used with the program.
A ' namespace' is typically a container for a collection of identifiers. A namespace provides a degree of context for different identifiers, making it possible to differentiate identifiers with the same name.
C++ transferred all the standard library's features to a namespace called "std" (standard).
The cout is the keyword used to print a message or a value to the user or on the screen.
The cin keyword takes input from the user.
A main is the default name given to the main function of a program where the return type means function should return some value, here return type int is given.
In a program, the return type of the main function is int, that is, it should return an integer value. The return is considered as the exit status of an application.
Sample Output:
Want to see more full solutions like this?
Chapter 4 Solutions
Programming and Problem Solving With C++
- C++ In this task, you are required to write a code that converts an Octal number into its equivalent Decimal value or vice versa. The user first selects the conversion choice by inserting 1 or 2. Choice 1 is followed by inserting an Octal integer and getting its Decimal equivalent value as an output. Choice 2 requires inserting a Decimal integer and prints out its Octal equivalent. The Octal numeral system, is the base-8 number system, and uses the digits 0 to 7, that is to say 10 octal represents 8 Decimal and 100 octal represents 64 Decimal. Convertion from an Octal integer into Decimal In the Decimal system, each number is the digit multiplied by 10 to the power of its location (starting from 0). For example: 74 decimal = 7 x 10^1 + 4 x 10^0 1252 decimal = 1 x 10^3 + 2 x 10^2 + 5 x 10^1 + 2 x 10^0 each number is the digit multiplied by 8 to the power of its location (starting from 0). For example: 112 octal = 1 x 8^2 + 1 x 8^1 + 2 x 8^0 Converting an Octal integer into…arrow_forwardA palindrome is a word that is spelled the same way both forwards and backwards. For example, the word, 'racecar' is a palindrome. Using character arrays, write a c++ program that prompts the user to enter a word from the keyboard and informs the user whether the word is a palindrome or not.arrow_forwardWrite a C++ program to calculate a rectangle's area. The program consists of the following function: getLength - This function should ask the user to enter the rectangle's length. and then returns that value as a double • getWidth - This function should ask the user to enter the rectangle's width. and then returns that value as a double. • get Area - This function should accept the rectangle's length and width as arguments and return the rectangle's area. • displayData - This function should accept the rectangle's length, width and area as arguments, and display them in an appropriate message on the screen. main - This function consists of calls to the above functions.arrow_forward
- Several websites will implement password rules. Write a C++ program that uses a function to check whether a string is a valid password. The password rule is as follows: - A password must have at least eight characters - A password consists of only letters and digits - A password must contain at least two digits Write a C++ program that prompts the user to enter a password and displays "valid password" if the rule is followed or "invalid password" otherwise. Sample 1: Enter a string for password: wewew43x valid password Sample 2 Enter a string for password: 343a Invalid password Document the following information and save it in a README file (Eg. Readme.txt) 1. Analysis: (Descibe the problem including input and output in your own words) Design: (Describe the major steps for solving the problem) Testing: (Describe how you test the program) You are required to submit: 1. The binary source code in C++ 2. The Readme filearrow_forwardFor C++ The first TODO is to gather the necessary input from the user to complete the story. Create the variables that you will need; be mindful of which data types to use! Remember that string input should use getline instead of just cin by itself. The second TODO is to display the story once again, but now with the values entered by the user. This is where you will use the variables you made previously. The third TODO is to seed the random number generator using the current system time. We will need this in order for the next section to work. The fourth TODO is to create a visual graph of the stock price fluctuating. You will need to use the setfill and setw stream manipulators to make this work. The stock price value determines how many of the symbols to display. Make sure to round the price to the nearest dollar so that the chart is as accurate as possible. There are five fluctuations that you need to model in your program: The stock price is multiplied by a random number between…arrow_forwardYou are working on a text mining project where you have to do some operations on text. Develop a C++ console application that does the following: Prompt for and get two strings from the user (each string has more than 10 characters). Print the length of each string. Convert the 5th character of the first string to an uppercase character and print it. Convert the 10th character of the second string to a lowercase character and print it. Output values should be printed with left and right justified label Look at the sample input and output and check your console with two different inputs.arrow_forward
- In C++: You are working for a software firm that developed a word processor. A common error users often make is that they forgot the caps locks on and print text like this: " tHIS IS A SAMPLE of an error", this usually happens at the beginning of a new section (i.e. right after a dot or in a new line). Write a program to detect this error and fix it. The input will be a long string on keyboard.arrow_forwardIn C++ Programming, write a program that calculates and prints the monthly paycheck for an employee. The net pay is calculated after taking the following deductions: Federal Income Tax: 15% State Tax: 3.5% Social Security Tax: 5.75% Medicare/Medicaid Tax: 2.75% Pension Plan: 5% Health Insurance: $75.00 Your program should prompt the user to input the gross amount and the employee name. The output will be stored in a file. Format your output to have two decimal places. A sample output follows: Bill Robinson Gross Amount: .......................... $3575.00 Federal Tax: ................................ $ 536.25 State Tax: .....................................$ 125.13 Social Security Tax: ................... $ 205.56 Medicare/Medicaid Tax: ......... $ 98.31 Pension Plan: .............................. $ 178.75 Health Insurance: .......................$ 75.00 Net Pay: ....................................... $2356.00 NOTE: The first column is left-justified, and the right column is…arrow_forwardQ1 /A chemical sensor works to read the pollution value in four industrial sites every four hours a day, so you want to write a program in C + + language that reads the pollution values in those sites and finds the rate of pollution in each site and the highest pollution rate?arrow_forward
- In C++ Programming, write a program that calculates and prints the monthly paycheck for an employee. The net pay is calculated after taking the following deductions: Federal Income Tax: 15% State Tax: 3.5% Social Security Tax: 5.75% Medicare/Medicaid Tax: 2.75% Pension Plan: 5% Health Insurance: $75.00 Your program should prompt the user to input the gross amount and the employee name. The output will be stored in a file. Format your output to have two decimal places. A sample output follows: Bill Robinson Gross Amount: Federal Tax: State Tax: Pension Plan: . Health Insurance: $3575.00 Social Security Tax: Medicare/Medicaid Tax:.........$ 98.31 Net Pay:...... $ 536.25 $ 125.13 $ 205.56 $ 178.75 $ 75.00 $2356.00arrow_forwardIn C programming language, if the first and the second operands of operator + are of types int and float, respectively, the result will be of type A. int B. float C. char D. long intarrow_forwardC++ only Upvote for your own work and correct answer/ Downvote for copying the answer from a different website. It must be in C++ and it must be a working code. Thank You. Assignment: Create a C++ program that can test the validity of propositional logic. Remember, a propositional logical statement is invalid should you find any combination of input where the PROPOSITIONAL statements are ALL true, while the CONCLUSION statement is false. Propositional Statements: If someone has a rocket, that implies they’re an astronaut. If someone is an astronaut, that implies they’re highly trained. If someone is highly trained, that implies they’re educated. Conclusion Statement: A person is educated, which implies they have a rocket. Your output should declare the statement to either be valid or invalid. If it’s invalid, it needs to state which combination of inputs yielded the statement invalid. MAKE SURE IT POINTS OUT EVERY TIME THE STATEMENT IS INVALID IF IT IS INDEED INVALID.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
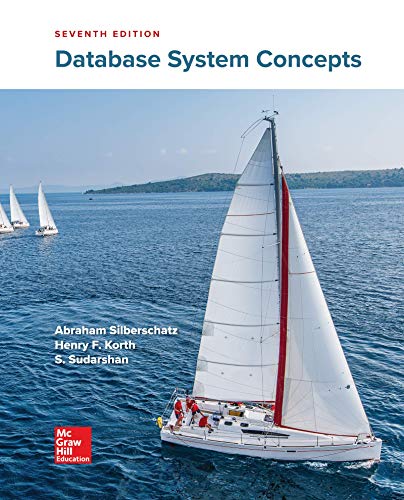
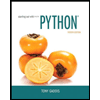
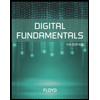
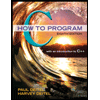
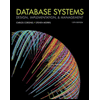
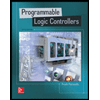